Java 반복문
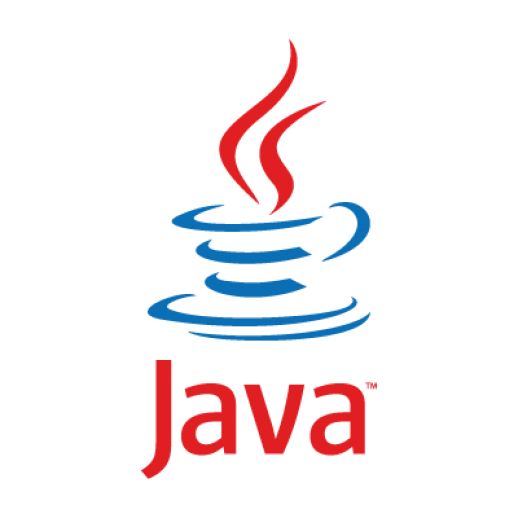
참고서적 : Java의정석 3rd Edition
do-while문
while문의 변형으로 기본적인 구조는 같으나 조건식과 블럭 {}의 순서를 바꿔놓은 것이다.
{}먼저 수행한 후에 조건식을 평가한다. 그러기에 최소한 한번은 수행될 것을 보장한다.
반복적으로 사용자의 입력을 받아서 처리할 때 유용하다.
랜덤한 값을 맞춰보세요.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| class example { public static void main(String[] args) { int input = 0, answer = 0; answer = (int)(Math.random() * 100); Scanner scan = new Scanner(System.in);
do{ System.out.print('1 ~ 100 정수입력'); input = scanner.nextInt();
if(input > answer) { System.out.print('더 작은 수 입력'); } else (input <= answer) { System.out.print('더 큰 수 입력'); } } while(input != answer); System.out.print('정답'); } }
|
먼저 input의 값을 입력받고 동작을 수행한 후에 while문의 조건을 확인하다.
while문이 input과 answer이 같을때 까지 반복임으로 같을때까지 반복한다.
3, 6, 9 구구단에 맞춰 “짝”을 출력하라!
1~100 중에 3, 6, 9가 포함되면 “짝”을 출력하라!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| package chapter04;
public class FlowEx29 {
public static void main(String[] args) {
for (int i = 1; i <= 100; i++) { System.out.printf("i=%d ", i); int temp = i;
do { if (temp % 10 % 3 == 0 && temp % 10 != 0) { System.out.print("짝"); } } while ((temp = temp/10) != 0); System.out.println(); } }
}
|
입력받은값 중에 3, 6, 9가 포함되면 “짝”을 출력하라!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| package chapter04;
import java.util.Scanner;
public class guguUpdate { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.print("값 입력 : "); int value = scan.nextInt(); while(value > 0) { if(value%10%3 == 0) { System.out.print("짝"); } value = value/10; } System.out.println("end"); } }
|
break문
break는 자신이 포함된 가장 가까운 반복문을 벗어난다.
숫자를 순차적으로 증가시켜 합쳤을때 그 sum이 100이 넘으면 break를 하라.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| package chapter04;
public class FlowEx30 { public static void main(String[] args) { int i = 0; int sum = 0; while(true) { if(sum > 100) { break; } i++; sum += i; } System.out.println("i = " + i); System.out.println("sum = " + sum); } }
|
while문안에 조건을 true로 넣었기 때문에 break 문이 없다면 무한루프가 될 것이다.
continue 문
continue문은 반복문 내에서만 사용되며 조건식이 참이되어 실행시 블럭의 끝으로 이동하여 다음 반복으로 넘어간다.
loop가 종료되는 것이 아니다.
for문의 경우 증감식으로 이동하며, while문과 do-while문의 경우 조건식으로 이동한다.
break VS continue
break는 반복문을 벗어난다.
continue 반복문 전체를 벗어나지 않고 다음 반복을 계속 수행한다.
메뉴를 보여주고 선택하게하며 잘못 선택한 경우 continue로 메뉴를 보여주고,
종료(4)를 선택한 경우 break문으로 종료한다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| package chapter04;
import java.util.ArrayList; import java.util.Iterator; import java.util.Scanner;
public class FlowEx32 {
public static void main(String[] args) { ArrayList<String> arr = new ArrayList<String>(); arr.add("햄버거"); arr.add("피자"); arr.add("치킨"); arr.add("밥"); Iterator<String> itr = arr.iterator(); while(itr.hasNext()) { String menu = itr.next(); System.out.println(arr.indexOf(menu)+" "+menu); } while (true) { System.out.print("choice Menu : "); Scanner scan = new Scanner(System.in); int select = scan.nextInt(); if(select >= arr.size()) { continue; } System.out.println(arr.get(select)); continue; }
} }
|
이름 붙은 반복문
break는 여러 개의 반복문이 중첩된 경우에는 break문으로 중첩 반복문을 완전히 벗어날 수 없다.
이때 중첩 반복문 앞에 이름
을 붙이고 break문과 continue문에 이름을 지정해 줌으로써 중첩 반복문을 벗어나거나 건너 뛸 수 있다.
아래는 간단한 예제이다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package chapter04;
public class FlowEx33 {
public static void main(String[] args) { for (int i = 2; i <= 9; i++) { for(int j = 1; j <= 9; j++) { System.out.println("구구단 : "+i+"단 = "+i+"*"+j+"="+i*j); if(j == 5) break; } } } }
|
따로 중첩 반복문에 이름을 지정하지 않고 j == 5 일경우에 break를 했더니 아래처럼 9단까지 전부다 출력이 된다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| 구구단 : 2단 = 2*1=2 구구단 : 2단 = 2*2=4 구구단 : 2단 = 2*3=6 구구단 : 2단 = 2*4=8 구구단 : 2단 = 2*5=10 구구단 : 3단 = 3*1=3 구구단 : 3단 = 3*2=6 구구단 : 3단 = 3*3=9 구구단 : 3단 = 3*4=12 구구단 : 3단 = 3*5=15 구구단 : 4단 = 4*1=4 구구단 : 4단 = 4*2=8 구구단 : 4단 = 4*3=12 구구단 : 4단 = 4*4=16 구구단 : 4단 = 4*5=20 구구단 : 5단 = 5*1=5 구구단 : 5단 = 5*2=10 구구단 : 5단 = 5*3=15 구구단 : 5단 = 5*4=20 구구단 : 5단 = 5*5=25 구구단 : 6단 = 6*1=6 구구단 : 6단 = 6*2=12 구구단 : 6단 = 6*3=18 구구단 : 6단 = 6*4=24 구구단 : 6단 = 6*5=30 구구단 : 7단 = 7*1=7 구구단 : 7단 = 7*2=14 구구단 : 7단 = 7*3=21 구구단 : 7단 = 7*4=28 구구단 : 7단 = 7*5=35 구구단 : 8단 = 8*1=8 구구단 : 8단 = 8*2=16 구구단 : 8단 = 8*3=24 구구단 : 8단 = 8*4=32 구구단 : 8단 = 8*5=40 구구단 : 9단 = 9*1=9 구구단 : 9단 = 9*2=18 구구단 : 9단 = 9*3=27 구구단 : 9단 = 9*4=36 구구단 : 9단 = 9*5=45
|
이러한 상황을 해결하고 싶다면 중첩반복문에 이름을 지정해주면 된다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| package chapter04;
public class FlowEx33 {
public static void main(String[] args) { Loop1 : for (int i = 2; i <= 9; i++) { for(int j = 1; j <= 9; j++) { System.out.println("구구단 : "+i+"단 = "+i+"*"+j+"="+i*j); if(j == 5) break Loop1; } } } }
|
중첩반복문에 Loop1이라는 이름을 붙여주고 break Loop1을 함으로써 구구단은 2단의 5번째까지만 출력된다.
1 2 3 4 5
| 구구단 : 2단 = 2*1=2 구구단 : 2단 = 2*2=4 구구단 : 2단 = 2*3=6 구구단 : 2단 = 2*4=8 구구단 : 2단 = 2*5=10
|
break
만 사용하면 해당 반복문을 빠져나가고 break 이름
하면 묶인 반복문을 전부다 빠져나간다.